When delving into the realm of React and exploring Drag and Drop libraries, key questions might emerge: Which React Drag and Drop libraries reign in popularity? How do I pinpoint the perfect library for my project? And what features are essential in a React Drag and Drop library?
Drag and drop stands as a user-friendly interface concept, enabling swift and straightforward reorganization of page elements. This functionality has become a staple in numerous web applications, prompting React developers to craft a variety of open-source libraries to facilitate the integration of drag-and-drop features in React projects.
This article is designed to equip you with a comprehensive grasp of the React Drag and Drop libraries at your disposal, ensuring you make an informed selection suited to your project’s needs. Additionally, you will acquire knowledge on how to seamlessly integrate and commence utilizing your chosen React Drag and Drop library. This insight is particularly valuable in the development of business software, where intuitive UI components like drag and drop can significantly enhance user experience and operational efficiency.
What is Drag and Drop?
Drag and drop, a user interaction technique allowing the movement of elements within the UI is foundational to numerous well-regarded applications like Trello, Jira, Zapier, and Monday. In these platforms, drag and drop serves as a core functionality, enabling users to reposition items effortlessly.
Beyond enhancing the modern user experience, this interaction style is particularly beneficial for individuals facing challenges with manual actions or those with disabilities, making digital environments more accessible and navigable.
The development of a smooth, efficient drag-and-drop feature, however, is not as straightforward as it might seem. Crafting high-quality, clean, and functional JavaScript implementations requires significant coding effort. This is why many developers opt for ready-made libraries, which offer pre-written code snippets that expedite the development process. Utilizing these libraries not only saves time but also ensures the incorporation of a polished and user-friendly drag-and-drop feature into web applications, an essential aspect in the development of intuitive and inclusive business software.
Drag and Drop Types
There are dozens of open-source libraries that help to create draggable and movable elements (e.g. lists, cards, tables, etc) in your React app. And, this option can simplify the UX route, in comparison to the process of filling in forms, and shortens the time of one or another formal action.
The most common use cases for drag-and-drop in React include uploading files; replacing the components within created lists and rearranging images and assets.
Basic Concepts
DragDrop Container: where you hold and then take out the component (data)
Children: the content of dataItem;
DragDropContext: is the place where drag-and-drop is carried out;
Droppable: the component that allows draggable components to be able to drop at the targeted area;
Draggable: the component which will be replaced;
As well as Droppable, it requires several properties to make the component displaceable;
onDragStart: The onDragStart component occurs when the user starts to drag an element;
onDragEnd: the component known as DragEnd occurs after the action has been accomplished;
DataTransfer: the component that can hold data about the dragged object;
DropTarget: a component that contains drop data;
How to Choose a Good Drag and Drop? 🤔
Surely, this is a relatively controversial question, because if you have enough time at your disposal, you may start coding on your own. Moreover, if you’re a junior programmer, we would not recommend that you use any ready libraries, but try to figure out the problem using your code. Yes, bugs are inevitable, though after each challenge there will surely be progress.
Typescript vs. Javascript Library
The vast majority of drag-and-drop libraries are written with the help of Typescript prevalence because Typescript is known for being structured, highly secure, and more consistent than vanilla Javascript. At the same time, it takes longer to compile the code in TypeScript, whereas JavaScript is more easy and flexible in terms of development.
So, if you are an old-school adherent, who is devoted to JS, you should understand that you need to know Typescript peculiarities to write with it. Plus, the size of the code will increase, because Typescript requires extremely detailed and accurate coding.
🤖 How to Build Custom Draggable Components in React?
To enable dragging on the component we need to proceed with this path:
- First off, create a drag component (drop area), in other words — container, where you will further drag
dataItem
. - Set the draggable attribute on the
dataItem
- Handle
onDragStart
event - Add here
event.dataTransfer.setData
event.dataTransfer.setData
component will contain some data, dataItem- Create a function
startDrag
event - Create a dropTarget component; it will call an event handler when dataItem with children will be dropped in
- Handle
onDragOver
event - Create
event.preventDefault
() function that will enable the dropping process of the component - Handle
onDrop
event - Set the consent function –
getData
- Call the dropped component
onItemDropped
- Finally, return the components to their initial state,
<div onDragOver={dragOver} onDrop={drop}> {props.children}
</div>);
Voila! This way your component will be ‘transferred’ from the container to the DropTarget.
How to Make Drag and Drop With React dnd library?
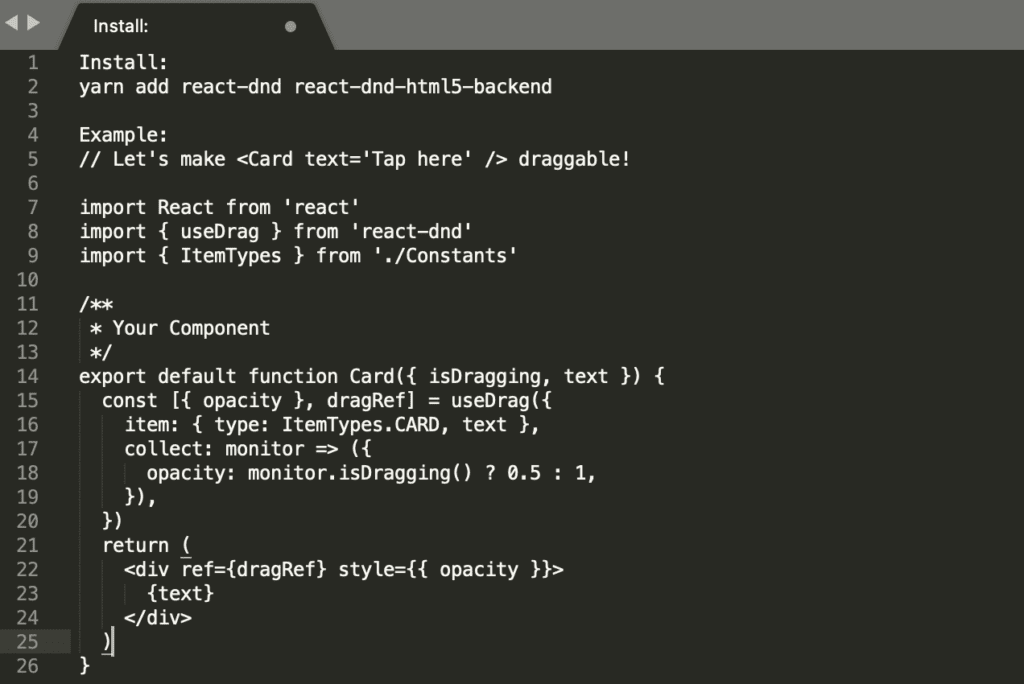
React Drag’n’Drops Libraries List
1. React Beautiful Dnd
React Beautiful dnd is the most popular library to build React Drag and Drop lists easily. It has already won the hearts of 23.8k developers on GitHub, thanks to its high performance. It has a clean and powerful API that is easy to work with and it fits well with any modern browser.
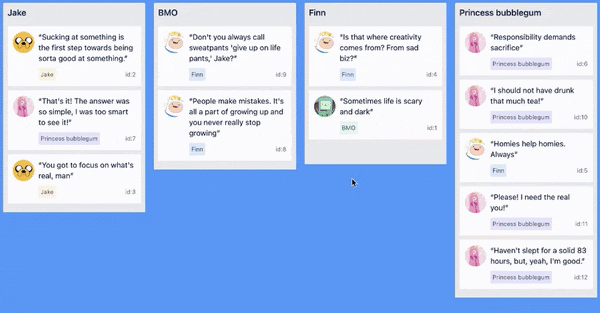
2. React Drag and Drop Container
Here the name of the library speaks for itself. React Drag Drop container offers functionality for mouse and touch devices; i.e. it allows you to set up a draggable element, and drop a target for it, plus, it highlights the drop target when dragging over it (highlightClassName
). Also, you can drag an element copy of the element, or let the element disappear while dragging (disappearDraggedElement
).
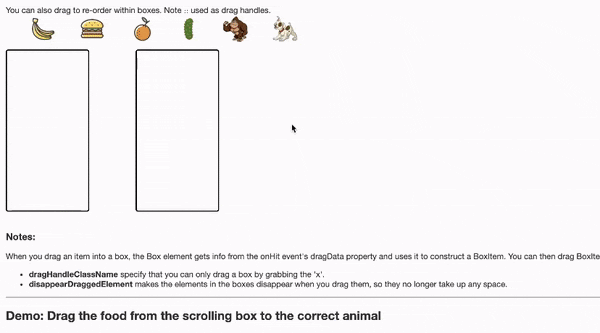
3. Draggable
Another well-deserved library does not perform any sorting behavior while dragging, it has the following modules: Droppable, Sortable, and Swappable. Draggable itself does not perform any sorting behavior while dragging, but does the heavy lifting, e.g. creates a mirror, emits events, manages sensor events, and makes elements draggable.
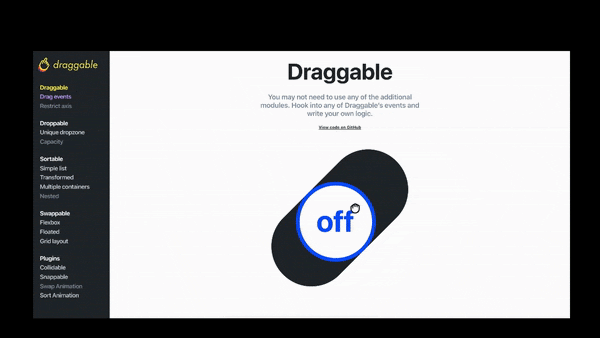
4. React Grid Layout
React Grid Layout library has 13,5k ⭐ stars on GitHub. React Grid Layout has an ascetic design with static widgets, draggable and resizable widgets, and it supports breakpoints. You can drag the elements, and you can resize them. The closest similar instrument is called Packery, though this is a bin-packing layout library using jQuery, which React Grid Layout doesn’t use.
➕: React-Grid-Layout works well with complex grid layouts that require drag-and-drop, such as dashboards which can be resized(e.g., looker, data visualization products, etc.)
➖: Because of the clunky API, React-Grid-Layout may need more calculations and it’s a better fit for large-scale apps.
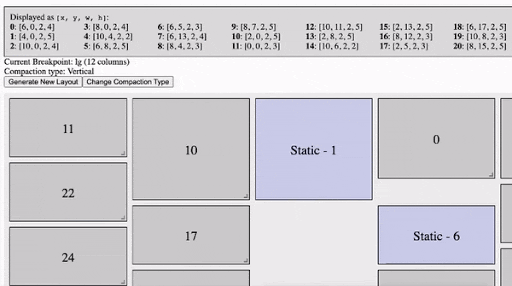
5. React Dropzone
React Dropzone is an example of a simple HTML5 drag-and-drop zone with React.js. It requires a standard installation process with the npm command and using a module bundler like Webpack or Browserify. React Dropzone has 8.2 ⭐ Github stars and is based on Javascript.
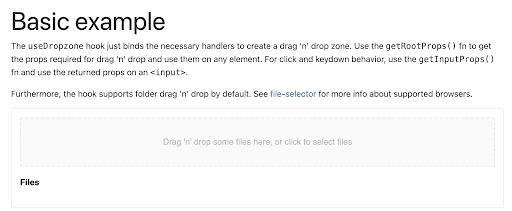
6. React DND
React DND got 15.9k ⭐ stars on GitHub, and was written mainly with the help of TypeScript, as well as JavaScript and CSS. It has the sort option, group option, delay option, delayOnTouchOnly option, swapThreshold option, and many other essential features for implementing drag-and-drop components. React DND works well with grids, and one-dimensional lists, but it’s harder to work with than for instance the well-known react-beautiful-dnd when you need to customize something individually.
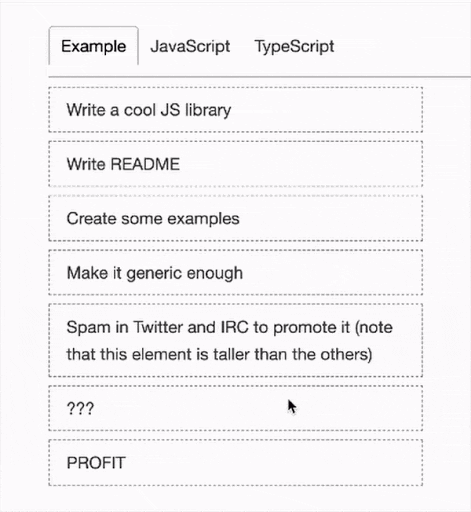
7. React SortableJS
React sortable is one more brilliant instrument made with Javascript and HTML, commonly used for creating drag-and-drop lists. It has all the basic functionalities of sorting/delaying/swapping/inverting and lots of others. Available on all touch modern browsers and touch devices.
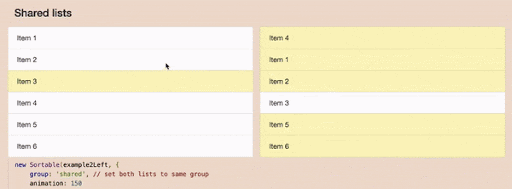
8. Interact.js
Snap, resize and customize your drag-and-drop elements with Interact.js. The library has also an advanced version, check it out here. It also supports evoking simultaneous interactions; and interaction with SVG and works well with desktop and mobile versions of Chrome, Firefox, and Opera as well as Internet Explorer 9+. Sharp has 10.2 k ⭐ on GitHub and
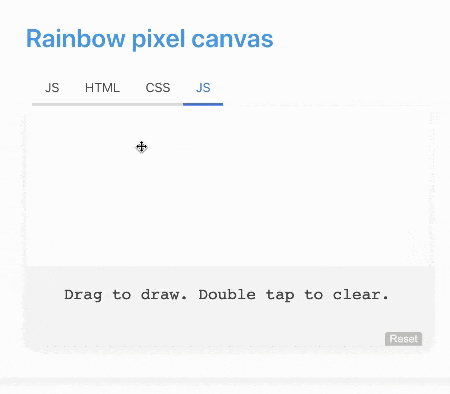
9. React Kanban
React Kanban is a relatively young but super pluggable library positioning itself as ‘one more Kanban/Trello board lib for React’. Kanban was written with the help of JavaScript, SCSS, and HTML. But, be careful using this library with lots of cards (more than 1k), cause then you may face some performance hurdles. In this case, virtual lists may become the solution.
10. Juggle and Drop
Juggle and Drop is an instrument to manage your tasks made with pure Javascript, with the help of React, redux, MLAB, express Mongodb, and Google Analytics. With Juggle and Drop you can add, edit, and delete cards and lists; clone the component, navigate to the root directory, and others.
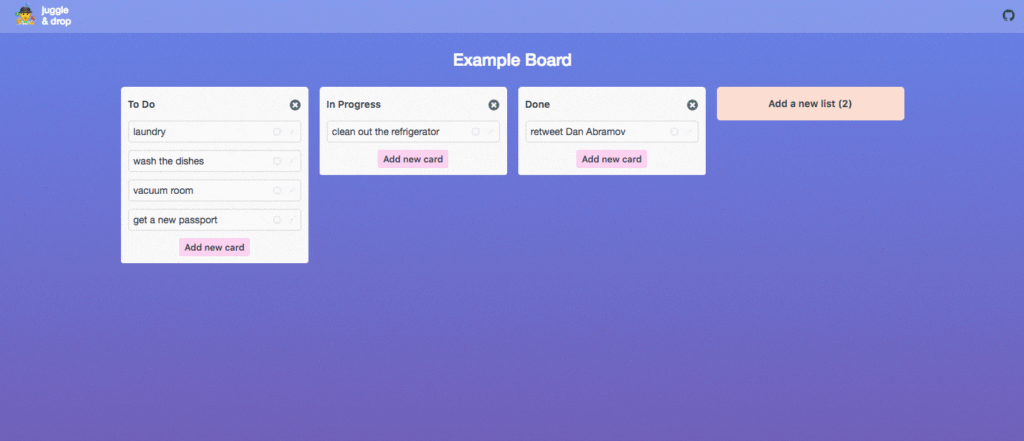
11. React Motion
This one more a highly recommended and really powerful package for working with animation functions in JS. This package has 19.7k ⭐ on GitHub, and 612,446 installations according to NPM. Among others, it has sorting lists with drag and drop. How to get started? npm install
— save react-motion and keep working!
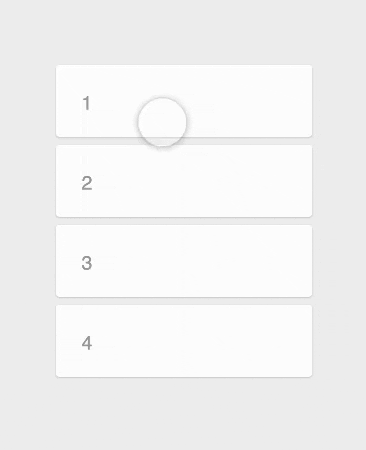
12. React Smooth DnD
The React-smooth drag-and-drop package is a super lightweight and highly customizable library for React with 1.5k stars on GitHub and with lots of configuration options covering D&D scenarios. The cardboard and fonts look neat and pleasant to the eye.
13. Nested DND
Nested DND in juicy colors helps to drag a part of the stack with the items lying on top of the dragged one. Drop it on top of any plays, and see how simple and intuitive it is.
14. React Nestable
React Nestable, an example of a JavaScript drag-and-drop library, is a drag-and-drop hierarchical list made with a neat bit of deduction. This package is finalizing our list of open-source drag-and-drop libraries recommended while building the dnd option.
15. React Files Drag and Drop
One more relatively fresh library to manage and customize your inner drag-and-drop component easily is React-files-drag-and-drop. It has a list of basic properties and was developed with TypeScript and CSS language.
Check more examples of React drag and drop on codesandox or here.
Wrapping Up
Now you know enough about React DnD libraries and it is high time to explore further the rest of the documentation in detail! Stay motivated, don’t be afraid of making mistakes, and debugging them! Well, this is a good place to tell: if you’ve never made a mistake, you’ve probably never done anything.
About Flatlogic
At Flatlogic, we carefully craft dashboard templates on React, Vue, Bootstrap, and React Native to bootstrap coding. We are mentioned in the Clutch list of top-performing agencies from all over the world. In the last 6 years, we have completed more than 50 templates and large-scale custom development projects for small startups and large enterprises. We love our work and know that only beauty (beautifully designed templates 🙂 ) can save the world.
Bonus: Creating Applications with Flatlogic Platform
At Flatlogic, we have developed a modular tool for creating web applications within minutes and with minimum expertise. Flatlogic platform doesn’t need you to code or write technical specifications. Instead, you only have to make a few choices and let the Platform compile the App for you.
#1: Name your project
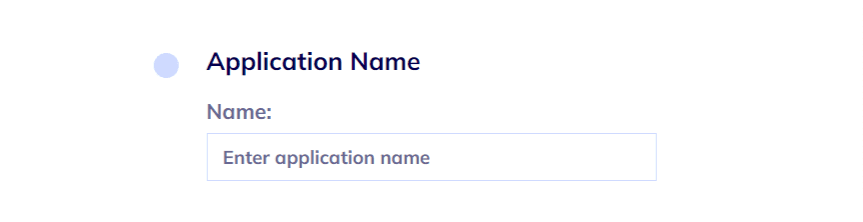
First of all, choose a name for your project that you’ll easily associate with it.
#2: Choose Tech Stack
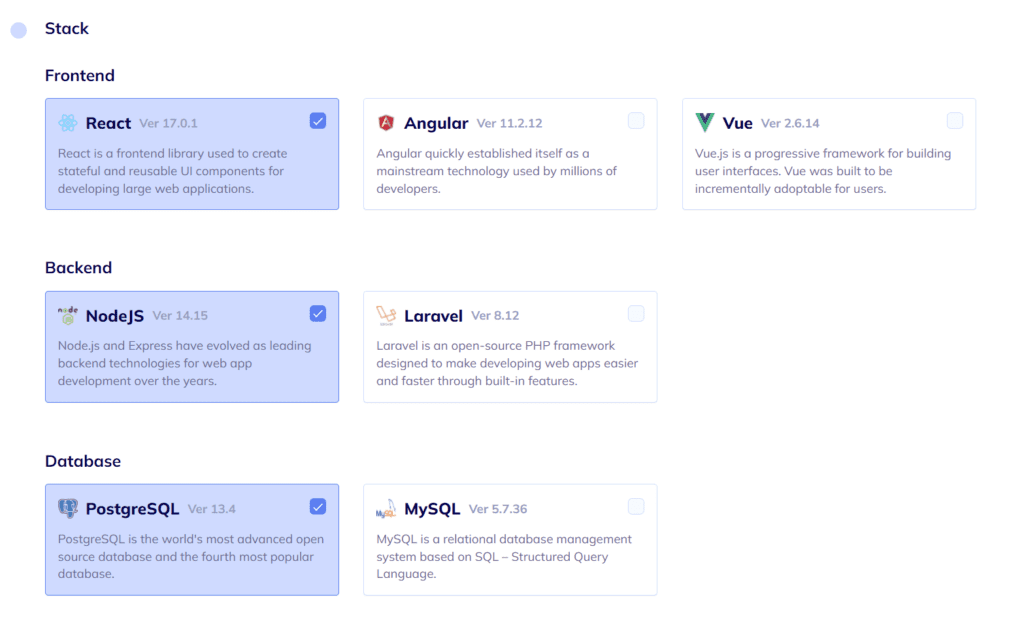
Choose underlying technologies for the front-end, the backend, and the database. In the example above we chose React, Node, and PostgreSQL, respectively.
#3: Choose the Design
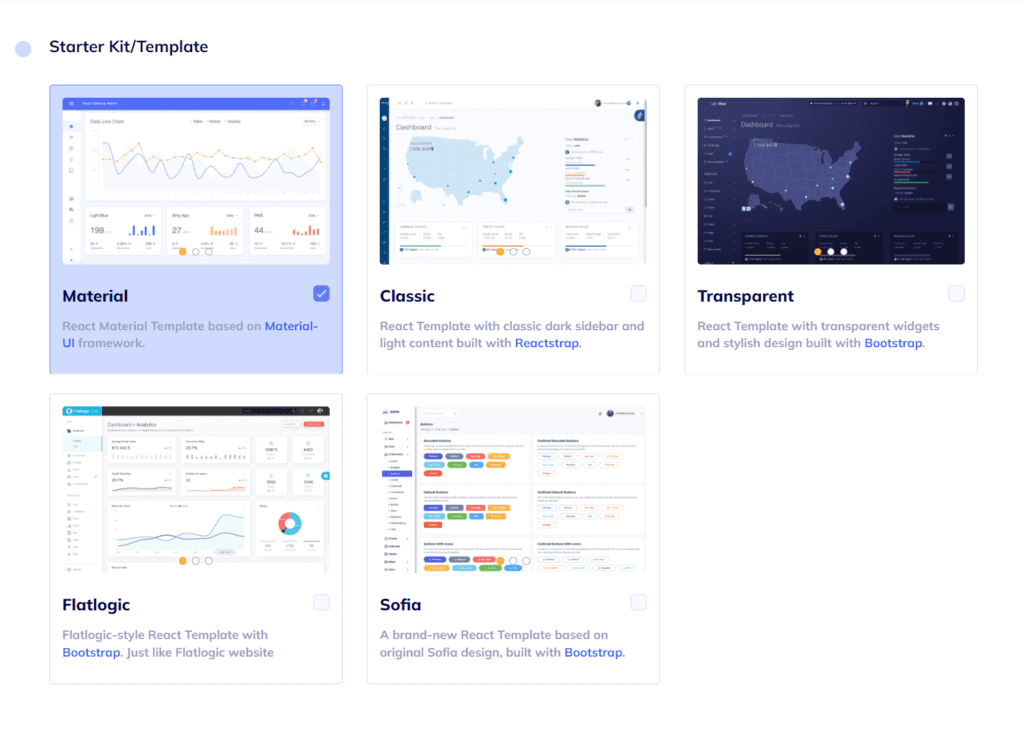
Define how your App’s interface will look. Flatlogic platform offers several distinct styles.
#4: Define the Database Schema
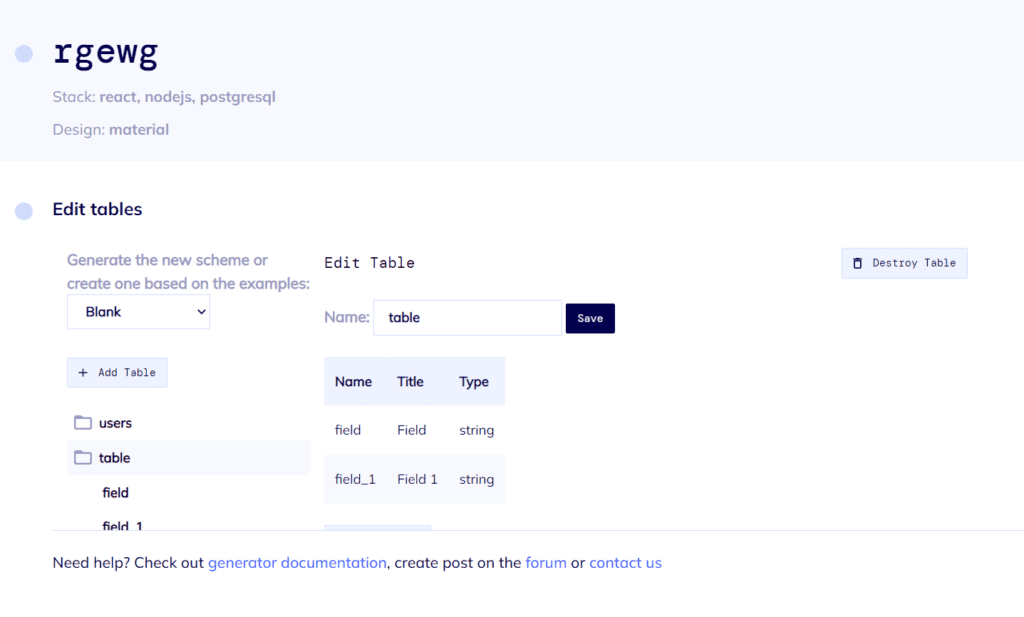
This is perhaps the hardest part. Define the fields, columns, and data types in your database. If it seems too difficult, you can choose one of the ready schemas we crafted for popular demands.
#5: Finish your App
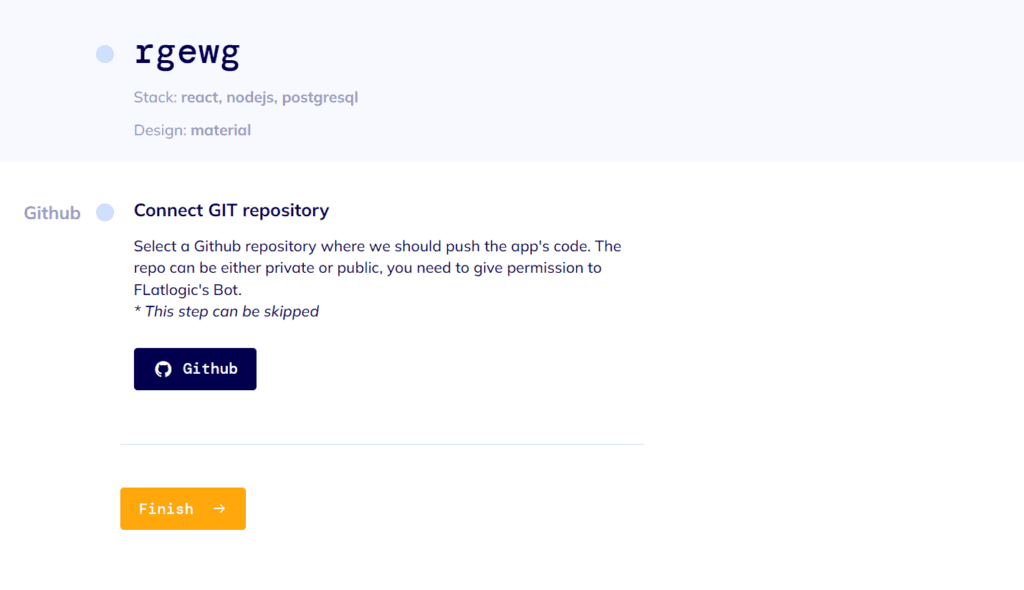
All the main choices have been made. Check if everything is as intended, check the “Connect GIT repository” box, and hit “Finish”. The Platform will compile for a couple of minutes and offer you your very own application. Enjoy!
Comments