Internationalization is an integral part of modern software development. In addition to English-speaking countries, there are still many paying markets where a project may not be successful without internationalization and localization. Different studies show that 72% of consumers are more likely to stay on a website if it has been translated. Moreover, 55% of consumers said they only buy from websites that are in their native language.
In this regard, the question arises of how to do internationalization at the software development level, namely in the React framework.
In this article, we will look at what internationalization is, how to do internationalization using the i18next library as an example, consider the best internationalization libraries for React, and also find out the difference between internationalization and localization.
What is Internationalization
Internationalization is the process of translating your application into different languages. Internationalization or i18n is the design and development of a product, application, or document content that enables easy localization for target audiences that vary in culture, region, or language.
Translation of the application includes many aspects, such as changing the date, language, position of letters, and the like. Unicode usage, legacy character encodings, string concatenation, etc. are some of the things which assist in designing an application to ensure successful internationalization.
Difference between internationalization and localization
Software localization is different from internationalization (i18n). Internationalization covers areas such as software development and design across different cultures and languages. In other words, internationalization is what allows localization to happen in the first place. Internationalization is typically done by software developers, while localization is done by translators.
Also, an important aspect of internationalization is instead of writing code for each language, you replace code with placeholders that automatically retrieve the correct language for the user without engineering changes.
With localization, you can not only customize the language preference but also number formats, date and time formats, currency formats, keyboard layout, sorting and collection, text direction, and even colors and designs.
Localization also can include the cultural and country-specific aspects of different languages, such as:
- Accounting standards;
- Links and other resources;
- Calendars;
- Hand symbols, gestures, or signage;
- Culturally appropriate images;
- Dates and times;
- Spelling and phrasing, such as the differences between the Spanish spoken in Argentina and Spain;
- Right-to-left languages like Arabic or Hebrew.
React Internationalization guide
Base project
The basic project for us will be a simple page of text with personal information in two languages – German and English, with text switching by means of a button. We will develop our application using create-react-app.
Prerequisites
This tutorial assumes that you have installed the latest version of Node.js and npm or yarn on your device. Moreover, you need to have some experience with simple HTML, JavaScript, and basic npm and Yarn commands, before jumping to React i18n.
You can view the code for my application by following the following link on GitHub.
Getting Started
In this section, I will help you understand the integration between i18next and React. To make things a bit easier and smooth, we will make use of the Create React App package by Facebook.
The first step is to install the latest release of Create React App. To do this, open console/terminal depending on your operating system and enter the following command:
yarn create react-app [name of your app]
After the setup is complete, type this command in the console and run it.
cd [name of your app]
It will take you to the folder of the project.
Then run the following command to test if the application is installed correctly.
yarn start
If everything is working correctly you will see the working app on the localhost port.
Installing dependencies and making the actual application
Let’s install all required dependencies to develop our i18n application.
yarn add i18next react-i18next i18next-browser-languagedetector
The browser language detector automatically detects the language of your location.
We also use react-bootstrap
in our application, but it is optional.
Let’s create the content for the translation. In my case, it will be the information about me and the page look like my personal in app.js
file. The code will look like that:
import React from 'react';
import Container from 'react-bootstrap/Container';
import Jumbotron from 'react-bootstrap/Jumbotron';
import Row from 'react-bootstrap/Row'
import Col from "react-bootstrap/Col";
import me from './me.jpg'
function App () {
return (
<Container>
<Jumbotron>
<Row>
<Col>
<p>I am a Product Owner at Flatlogic.com. The company creates templates on top of Javascript technologies and help to integrate these dashboards into existing products. During 3 years we grow our customer base over the 70k users and have almost 100k monthly visitors on our website.</p>
<p>Previously I was a co-founder of Kuoll, an error analytics platform for e-commerce. Together with my partner, I went from MVP to the first paid users and increased the user base from 100 to more than 750, which included learning programming, product management, marketing and sales, user support.</p>
<p>Before founding Kuoll I was an Audit Staff at Ernst & Young where I helped analyze data and business, perform general substantive audit procedures for Fortune 5000 companies.</p>
<p>I was fortunate enough to be alumni of one of the largest startup accelerators in Nordic and Baltic states, Startup Sauna and Starta Accelerator in New York. Now, I am also receiving BA in Computer Science at University of London. I also love running, cycling, my wife Yuliya and daughter Sasha.</p>
</Col>
<Col>
<img src={me} alt="Eugene Stepnov" id="me" />
</Col>
</Row>
</Jumbotron>
</Container>
)
}
export default App;
The components like <Jumbotron>
, <Container>
and others are from react-bootstrap. This is a basic personal page.
Next, we will need to create a file where the text of our translation, the configuration will be stored, as well as the plug-in from i18next will be loaded, our file will be called translation.js and will look like this:
import i18n from 'i18next';
import {initReactI18next, Trans} from "react-i18next";
import LanguageDetector from 'i18next-browser-languagedetector';
import React from "react";
i18n
.use(initReactI18next)
.use(LanguageDetector)
.init({
// we init with resources
resources: {
en: {
translations: {
"I am a Product Owner at Flatlogic.com. The company creates templates on top of Javascript technologies and help to integrate these dashboards into existing products. During 3 years we grow our customer base over the 70k users and have almost 100k monthly visitors on our website." : "I am a Product Owner at Flatlogic.com. The company creates templates on top of Javascript technologies and help to integrate these dashboards into existing products. During 3 years we grow our customer base over the 70k users and have almost 100k monthly visitors on our website.",
"Previously I was a co-founder of Kuoll, an error analytics platform for e-commerce. Together with my partner, I went from MVP to the first paid users and increased the user base from 100 to more than 750, which included learning programming, product management, marketing and sales, user support." : "Previously I was a co-founder of Kuoll, an error analytics platform for e-commerce. Together with my partner, I went from MVP to the first paid users and increased the user base from 100 to more than 750, which included learning programming, product management, marketing and sales, user support.",
"Before founding Kuoll I was an Audit Staff at Ernst & Young where I helped analyze data and business, perform general substantive audit procedures for Fortune 5000 companies." : "Before founding Kuoll I was an Audit Staff at Ernst & Young where I helped analyze data and business, perform general substantive audit procedures for Fortune 5000 companies.",
"I was fortunate enough to be alumni of one of the largest startup accelerators in Nordic and Baltic states, Startup Sauna and Starta Accelerator in New York. Now, I am also receiving BA in Computer Science at University of London. I also love running, cycling, my wife Yuliya and daughter Sasha." : "I was fortunate enough to be alumni of one of the largest startup accelerators in Nordic and Baltic states, Startup Sauna and Starta Accelerator in New York. Now, I am also receiving BA in Computer Science at University of London. I also love running, cycling, my wife Yuliya and daughter Sasha."
}
},
de: {
translations: {
"I am a Product Owner at Flatlogic.com. The company creates templates on top of Javascript technologies and help to integrate these dashboards into existing products. During 3 years we grow our customer base over the 70k users and have almost 100k monthly visitors on our website." : "Ich bin Product Owner bei Flatlogic.com. Das Unternehmen erstellt Vorlagen auf Javascript-Technologien und hilft bei der Integration dieser Dashboards in bestehende Produkte. Während 3 Jahren haben wir unseren Kundenstamm auf über 70.000 Benutzer erweitert und haben fast 100.000 monatliche Besucher auf unserer Website.",
"Previously I was a co-founder of Kuoll, an error analytics platform for e-commerce. Together with my partner, I went from MVP to the first paid users and increased the user base from 100 to more than 750, which included learning programming, product management, marketing and sales, user support." : "Zuvor war ich Mitgründer von Kuoll, einer Fehleranalyseplattform für E-Commerce. Zusammen mit meinem Partner bin ich vom MVP zu den ersten kostenpflichtigen Nutzern aufgestiegen und habe die Nutzerbasis von 100 auf über 750 erhöht, was Lernprogrammierung, Produktmanagement, Marketing und Vertrieb, Nutzersupport beinhaltete.",
"Before founding Kuoll I was an Audit Staff at Ernst & Young where I helped analyze data and business, perform general substantive audit procedures for Fortune 5000 companies." : "Vor der Gründung von Kuoll war ich als Audit Staff bei Ernst & Young tätig, wo ich bei der Analyse von Daten und Geschäften sowie bei der Durchführung allgemeiner substanzieller Auditverfahren für Fortune 5000-Unternehmen half.",
"I was fortunate enough to be alumni of one of the largest startup accelerators in Nordic and Baltic states, Startup Sauna and Starta Accelerator in New York. Now, I am also receiving BA in Computer Science at University of London. I also love running, cycling, my wife Yuliya and daughter Sasha." : "Ich hatte das Glück, Alumni eines der größten Startup-Accelerators in den nordischen und baltischen Staaten zu sein, Startup Sauna und Starta Accelerator in New York. Jetzt erhalte ich auch einen BA in Informatik an der University of London. Außerdem liebe ich Laufen, Radfahren, meine Frau Yuliya und meine Tochter Sasha."
}
}
},
fallbackLng: 'en',
debug: true,
ns: ['translations'],
defaultNS: 'translations',
keySeparator: false,
interpolation: {
escapeValue: false,
formatSeparator: ','
},
react: {
wait: true
}
});
export default i18n;
Thus, in resources, you need to place your translation opposite each key.
Next, we need to update our root component to use this i18n config inside the index.js
:
Just import './translations';
Let’s get back to our main App.js
file to use our translation. The first step would be to import the i18next library.
​​import { useTranslation, Trans } from 'react-i18next';
Next, let’s add some variables regarding our translation and language switcher. Add this code to the App function:
const { i18n } = useTranslation();
const changeLanguage = (lng) => {
i18n.changeLanguage(lng);
};
And this code in return()
to change language:
<button onClick={() => changeLanguage('en')}>en</button>
<button onClick={() => changeLanguage('de')}>de</button>
The final step would be to wrap our text into the <Trans>
component which enables you to nest any react content to be translated as one string. Supports both plural and interpolation.
<p><Trans>I am a Product Owner at Flatlogic.com. The company creates templates on top of Javascript technologies and help to integrate these dashboards into existing products. During 3 years we grow our customer base over the 70k users and have almost 100k monthly visitors on our website.</Trans></p>
And that’s all, the final code for App.js
is looking now like this:
import React from 'react';
import Container from 'react-bootstrap/Container';
import Jumbotron from 'react-bootstrap/Jumbotron';
import Row from 'react-bootstrap/Row'
import Col from "react-bootstrap/Col";
import { useTranslation, Trans } from 'react-i18next';
import me from './me.jpg'
function App () {
const { i18n } = useTranslation();
const changeLanguage = (lng) => {
i18n.changeLanguage(lng);
};
return (
<Container>
<button onClick={() => changeLanguage('en')}>en</button>
<button onClick={() => changeLanguage('de')}>de</button>
<Jumbotron>
<Row>
<Col>
<p><Trans>I am a Product Owner at Flatlogic.com. The company creates templates on top of Javascript technologies and help to integrate these dashboards into existing products. During 3 years we grow our customer base over the 70k users and have almost 100k monthly visitors on our website.</Trans></p>
<p><Trans>Previously I was a co-founder of Kuoll, an error analytics platform for e-commerce. Together with my partner, I went from MVP to the first paid users and increased the user base from 100 to more than 750, which included learning programming, product management, marketing and sales, user support.</Trans></p>
<p><Trans>Before founding Kuoll I was an Audit Staff at Ernst & Young where I helped analyze data and business, perform general substantive audit procedures for Fortune 5000 companies.</Trans></p>
<p><Trans>I was fortunate enough to be alumni of one of the largest startup accelerators in Nordic and Baltic states, Startup Sauna and Starta Accelerator in New York. Now, I am also receiving BA in Computer Science at University of London. I also love running, cycling, my wife Yuliya and daughter Sasha.</Trans></p>
</Col>
<Col>
<img src={me} alt="Eugene Stepnov" id="me" />
</Col>
</Row>
</Jumbotron>
</Container>
)
}
export default App;
The application will work like this if everything runs smoothly.
Best internationalization libraries
Let’s take a look at the most well-known React i18n libraries. Before that, let’s see what options to use when looking for the right package for your app.
- Documentation and maintenance of the package;
- Feature / Library;
- Pluralizations;
- Nesting;
- How big is the community;
- Number Formats
- Date formats;
- Relative dates;
- HTML support;
- Performance/bundle size;
- Interpolation.
NPM Trends
Now let’s go directly to the overview of popular libraries.
Also, one of the important factors before starting to use the library is its popularity among other developers. As for the internationalization topic, judging by npm trends, the most popular library is i18next – let’s get ahead of ourselves and say that this is justified since the library is the easiest and most understandable to use.
i18next
I18next is an internationalization library that has been written for JavaScript and its frameworks. It provides a complete method for localizing the product as well as the other standard i18n features.
I18next is already a whole platform for managing the localization of your applications. The library has many plugins and integrations at its disposal, as well as a SaaS application for management. Some of these are a plugin to detect user’s language, loading & caching translations that might be handy for large scale apps.
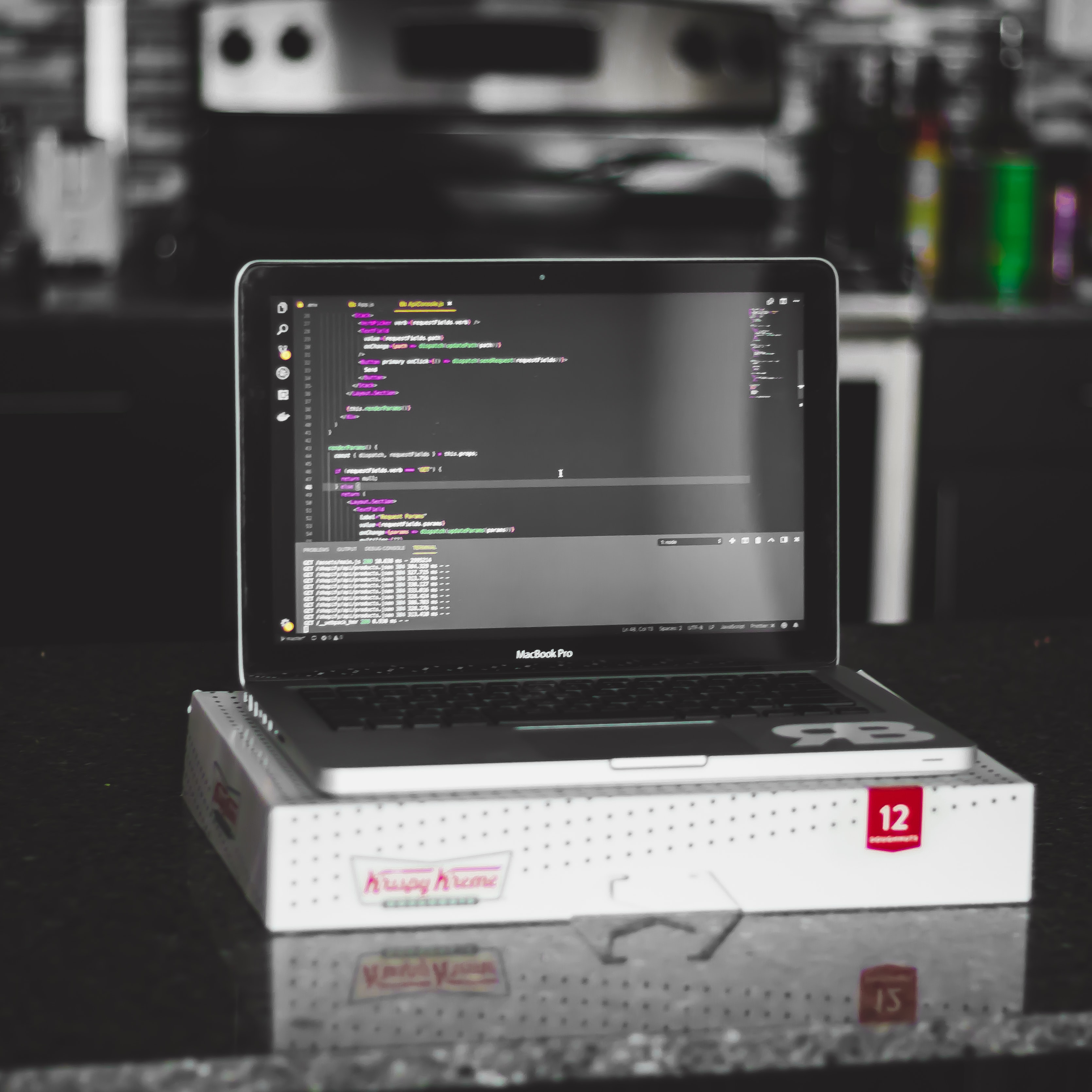
In Flatlogic we create web & mobile application templates built with React, Vue, Angular and React Native to help you develop web & mobile apps faster. Go and check out yourself!
See our themes!
One of the most important features of i18next is scalability. This allows the library to separate translations into multiple files and load them on demand.
The other good advantage of i18next over other libraries is sustainability, it was created in 2011 and is still constantly developed.
Features
- Highly effective and efficient API;
- Ecosystem;
- Maturity;
- Language detection;
- All tools to do complete localization.
Formatjs (React intl)
The react-intl library comes as a part of the FormatJS internationalization libraries. React-intl is one of the most popular libraries for the internationalization of React applications. It supports more than 150 languages globally. Library provides React components and an API to format dates, numbers, and strings, including pluralization and handling translations. It has a very larger community because it is the first i18n react library in the market.
React-intl provides react components and API to translate dates, numbers, currencies, and strings including pluralization.
However, the library has some disadvantages. You cannot use it for non-react components as it requires the top-level component to inject the context to the children.
React intl universal
React-intl-universal is developed by Alibaba Group and has all set of features to develop applications with internationalization. This library is one of the most popular solutions for i18n in React. It builds on top of React-intl and it enables even the non-React components to make use of the library by providing a singleton object to load the locale. For instance, this can be used in Vanilla JS as stated in the documentation itself.
Features
- Can be used not only in React.Component but also in Vanilla JS;
- Simple. Only three main API and one optional helper;
- Display numbers, currency, dates and times for different locales;
- Pluralize labels in strings;
- Support variables in message;
- Support HTML in message;
- Support for 150+ languages;
- Runs in the browser and Node.js;
- Message format is strictly implemented by ICU standards;
- Locale data in nested JSON format are supported;
- react-intl-universal-extract helps you generate a locale file easily.
Lingui JS
LinguiJS for React is the smallest of all i18n libraries available today for react applications. It uses ICU messages syntax and rich-text messages. Powerful CLI is included too to manage all the translation workflows.
Notable features
- Very small size;
- CLI;
- Good support from community;
- Well documented;
- Rich-text support.
React translated
React translated isn’t a very popular library, but very simple. The library is updating once a year.
Features
- Data interpolation;
- Component interpolation;
- Markdown inline-manipulations;
- Custom manipulations, pluralizations, and grammar rules based on input data;
- Component-level translation files (enables loading only required translations).
To translate the content of your app you just need to do two steps:
- Create a translation file that will contain a mapping of keys to the string in each language you support;
- Connect the Provider and wrap the top-level component with the <Provider> component according to props.
Conclusion
In this article, we got acquainted with the definition of internationalization, looked at how to make a simple application with translation using the i18next library, and also looked at the best libraries for internationalization.
If you liked the article, we would be happy if you share it with the world and leave us feedback.
Thank you!
Comments