Since there are several ways to send emails in Node.js, it’s reasonable to dive deeper into your options before sticking to a particular one. So how to proceed with it if you need to use your hosted SMTP server or build an HTML template? Let’s find out.
Options for sending emails in Node.js
One of the most popular methods of sending emails using Node.js is setting up an SMTP server to handle your outbound emails using Nodemailer, a Node.js module.
Another way is to send email using API. You can also connect multichannel notification services to support communication via other channels in a single place.
Besides general email-sending capabilities, these methods also provide other features, like bulk email, template building and deliverability rate tracking. But of course, each option works differently, hence providing both benefits and drawbacks.
So to figure out which method of email sending is the best fit for you, let’s dive into each of those deeper.
Sending emails with Node.js and SMTP
SMTP stands for Simple Mail Transfer Protocol and is one of the most widespread methods of sending emails. Emails sent via SMTP go through the following steps:
1. You compose an email, which is essentially a text file, in your email client, like Gmail or Outlook.
2. You click Send and the email is uploaded to your SMTP server for outgoing messages.
3. The server performs several stages of authentication to make sure you’re a safe sender and your message can be treated accordingly.
4. If it is authenticated successfully, the inbound SMTP server of your recipient’s email client receives the message, authenticates it and sends it to the user’s inbox
As you can see, the process is pretty straightforward, which is the reason behind its common use. But there are different sides to sending emails with Node.js and SMTP.
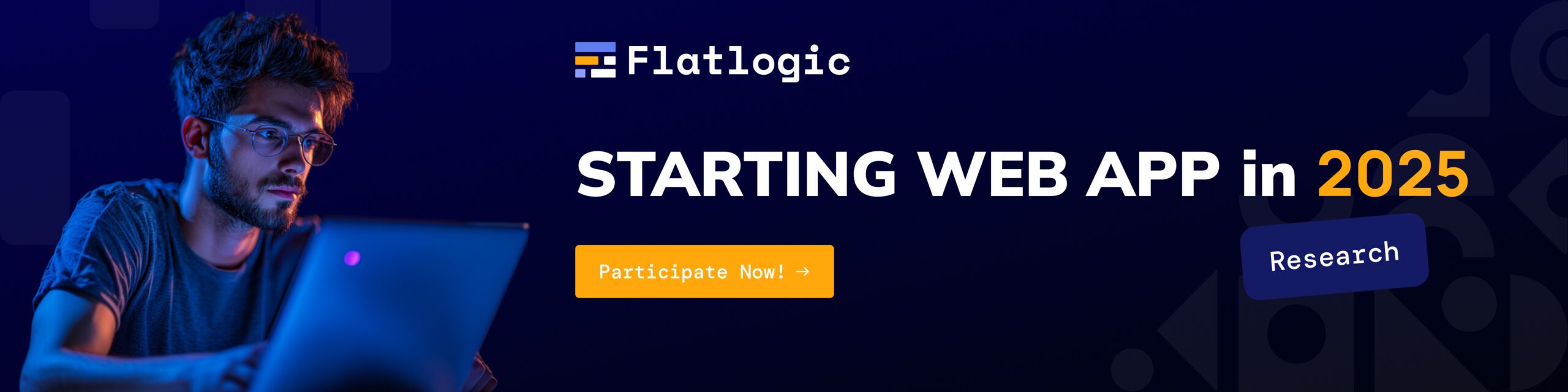
Image source: SocketLabs
Benefits of using SMTP
One of the main advantages of using SMTP to send emails through Node.js is that it is easy to integrate into a web application. By just indicating your server configurations as you code the app, you can set up an SMTP server for your outbound mail.
Another advantage of using SMTP is that your email address is protected from being blacklisted. The reason is that SMTP requires email address authentication. This means that your address is constantly checked whether you’re a spam sender to make sure your sender reputation remains positive and consistent.
Eventually, SMTP helps you keep your deliverability rate at a good point with effortless integration into your web app.
Disadvantages of using SMTP
As discussed above, an SMTP server uses several stages of authentication to verify the sender and the email. This makes the path of an email message from the sender to the recipient much longer than when using a transactional email API. And while this may not be a drastic difference for a single email, the time will add up when you send bulk campaigns.
Another drawback of using an SMTP server is that it will most likely be a frequent target of DDoS attacks, data breaches and phishing.
Of course, you can take measures to increase the security of your SMTP server, like constant server maintenance and long-term security monitoring. But not all organizations can afford ongoing server maintenance, so the risk of insecurity and cyber attacks remains a major disadvantage of this method.
Sending emails through Node.js and email API
A transactional email API is a service that allows sending emails using Node.js apps via an email API service. This means that you don’t have to host and maintain any servers on your own, like with SMTP. Instead, you use ready-to-go API from third-party providers.
There are many transactional email API services out there, from Amazon SES to Mailtrap Email API and SendGrid. While each service offers different features and functionality, they all are great assistants for managing email sending and deliverability.
Naturally, this method of sending emails with Node.js has both advantages and drawbacks.
Image source: GetResponse
Benefits of using a transactional email API
There are different advantages to using transactional email services. First of all, they usually come with a lot of documentation, so it’s extremely easy to set the API up in your Node.js application and start using it.
They also offer better security because you can use API keys, which reduces maintenance demand for this solution when compared to using SMTP. As a result, you get a secure way of email sending with lower engineering resources.
Besides, transactional email APIs offer diverse functionality for email management. You get extensive analytics to build reports on how your emails are delivering, what bounce and open rates are and other metrics. In the long term, this translates into actionable insights into your email marketing.
And speaking of long-term, transactional email API services offer great scalability opportunities. APIs allow sending high-volume campaigns, which become a must as your business expands.
Disadvantages of using a transactional email API
Despite all the benefits, there are some significant disadvantages to using a transactional email API to send your emails with Node.js.
One of the drawbacks is that you have to completely rely on a third-party service provider. While this may be helpful for troubleshooting, the dependence may pose a security risk for your data. Besides, you can’t control the service’s performance in case any issues occur on the provider’s end.
Another drawback of using this method is that you have to integrate different channels separately to set up notifications for the users.
For instance, if you want to set up SMS and chat app notifications along with push ones, you will have to configure each of the channels additionally. This means that more engineering time and effort have to be involved, which may not always be worth it.
Of course, you should do your research before choosing the provider of your transactional email API to choose a reliable one. Make sure to consider the features and guarantees they provide, as well as the deliverability rates and API documentation they offer.
Sending emails in Node.JS and multi-channel notifications services
Multi-channel notification services enable sending notifications across various channels, such as email, SMS, Slack, etc. via a single platform. They allow you to streamline your communication with the audience and notify them in the ways they find convenient.
Some notification services allow bringing your own email transaction API service or sending emails via an SMTP server so that you can manage all channels in one place. You can also get features like workflow creation and real-time delivery status.
Benefits of using multi-channel notifications services
The major advantage of using a multi-channel notification service for sending emails with Node.js is that you don’t have to add any new code or edit the existing one to add or remove channels.
Instead, you can just add those to the service’s settings and start using the new channel immediately. This means no time and effort to change up your multi-channel notification set-up, which makes it effortless to use.
Also, non-tech users can adjust their settings and change the look of an email in the app with no need to dive deep into how everything works. The same goes for analytics and email preview.
Lastly, you can use the same email transactional API for different channels, which makes multi-channel notification services a great addition to the API email sending.
Disadvantages of using multi-channel notifications services
The main drawback of using the service is the same as using API — you have to rely on a third party for the app’s performance. While this can be convenient and easier, this can also come with a number of risks, like the service’s security, speed and availability.
Sending emails in Node.js with Nodemailer
There are many modules you can use to send emails via Node.js since it is a server-side tool. But Nodemailer is the easiest module to install and use for email sending.
Nodemailer also provides a lot of features you’ll need to send different kinds of emails. From the support of HTML emails and attachments to the enabled use of SMTP, you get a lot of opportunities to customize your email sending.
To start sending emails with Node.js and Nodemailer, you have to install the module first. Follow the next steps to do that.
Step 1. Create your application in Node.js by running the following in the terminal:
mkdir nodemail-smtp
cd nodemail-smtp
npm init -y
You can skip this step and start with the next one if you have already created an app.
Step 2. Install the module using npm, which manages packages for Node.js, by writing the following command in the terminal:
npm install nodemailer
This will install the latest version of the Modemailer module. If the installation is successful, you’ll get a message similar to the following:
added 1 package, and audited 2 packages in 2s
found 0 vulnerabilities
Step 3. Now, we can start coding the app by importing it into your Node.js application:
const nodemailer = require('nodemailer');
Step 4. Create a Nodemailer transporter object using the createTransport method:
const transporter = nodemailer.createTransport(transport[, defaults]);
This method accepts an object with the configurations you set, like SMTP configurations, and returns a transporter object, which will be required to send emails later.
Next, we are going to use Gmail to show an example of how to send an email using Node.js and Nodemailer, but you can integrate other email clients, too. Since Gmail doesn’t allow direct email sending via Node.js because of security concerns, there are 2 solutions:
- making the Gmail account less secure
- using Oauth2 security authentication
1. Making the Gmail account less secure. You can access the Less secure account configuration by visiting the page and switching the toggle to ON.
This now allows creating the transporter object we started in Step 4 with the credentials to our Gmail account. To do this, run the following:
const nodemailer = require('nodemailer');
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: secure_configuration.EMAIL_USERNAME,
pass: secure_configuration.PASSWORD
}
});
Of course, you have to replace the credential values (user and pass) with valid ones.
2. Using Oauth2 security authentication. To set up this authentication method, we have to get the following values:
- Client ID
- Client Secret
- Refresh token
- Access token
- Credentials (username and password)
The next steps will guide you on how you can do this.
A. Go to Open Google Cloud Console and sign up (or sign in), then go to API & Services to create a project.
B. Register your application in the OAuth consent screen, choose the External user type and add a Test user.
C. Go to the Credentials selection page to create credentials, specifying Web application as the type of your app. Also, add a redirect URL as OAuth Playground from this list.
Great, you now have your Client ID and Client Secret available, as well as user credentials. Let’s get the rest of the data we need
D. Open the Oauh2 Playground to get Refresh and Access tokens. Go to Configuration and check the ‘Use your own credentials’ box. Insert the Client ID and Client Secret you got from the Cloud Console in Step C.
E. Select Gmail API and authorize it with the credentials of the Test user you added in Step B.
F. Get your Refresh and Access tokens by clicking on the Exchange authorization code for tokens.
Now, we have the correct values to use in our transporter object:
const nodemailer = require('nodemailer');
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
type: 'OAuth2',
user: secure_configuration.EMAIL_USERNAME,
pass: secure_configuration.PASSWORD,
clientId: secure_configuration.CLIENT_ID,
clientSecret: secure_configuration.CLIENT_SECRET,
refreshToken: secure_configuration.REFRESH_TOKEN
}
});
Step 5. Before actually sending an email message, you have to set up email configurations. This includes specifying what you are sending, to whom, etc. These configurations are set up using key-value pairs, like in the following example:
const mailConfigurations = {
from: '[email protected]',
to: '[email protected]',
subject: 'Sending Email using Node.js',
text: 'Hi! There, You know I am using the'
+ ' NodeJS Code along with NodeMailer '
+ 'to send this email.'
};
If you need to send an email to several receivers, just separate the receivers with a coma:
to: ‘[email protected], [email protected]’,
And there you go — you are ready to send your simple email via Nodemailer. To do that, you can use the sendMail() method.
Using the sendMail() method requires a transporter and a configured message, which we have already created in the previous steps. So we can send our email by running the following:
transport.sendMail(mailConfigurations, (error, info) => {
if (error) {
return console.log(error);
}
console.log('Message sent: %s', info.messageId);
});
Of course, you will get a notification if the message has been sent successfully, as well as you’ll get an error alert if something goes wrong.
How to send emails with SMTP
If you want to send your emails via your hosted SMTP server, you can easily set it up using the Nodemailer module. To do that, stick to the steps below.
Step 1. We assume that you have already created your Node.js app and installed Nodemailer. But you haven’t run the following in the terminal to create your app:
mkdir nodemail-smtp
cd nodemail-smtp
npm init -y
And to install Nodemailer:
npm install nodemailer
Step 2. Now, create a “nodemailer-smtp.js” file and fill it in with the following:
const nodemailer = require("nodemailer");
async function main() {
let info = await transporter.sendMail({
from: '"James Swanson" <[email protected]>',
to: "",
subject: "I love SMTP!",
text: "Here's a text version of the email.",
html: "Here's an <b>HTML version</b> of the email.",
});
console.log("Message sent: %s", info.messageId);
console.log("View email: %s", nodemailer.getTestMessageUrl(info));
}
main().catch(console.error);
Note that at this point, you need to have your SMTP server’s credentials ready so that you can set up the configurations in your code. In particular, you need the following:
- Server address
- Port
- Credentials for the server (username and password)
Next, we’re going to fill in this SMTP information in our code.
Step 3. Go to your SMTP configuration, copy the info from the list above and insert it into the async function main() {} object in your code. You’ll get something like this:
const nodemailer = require(“nodemailer”);
async function main() {
const transporter = nodemailer.createTransport({
host: “smtp.ethereal.email”,
port: 587,
auth: {
user: “[email protected]”,
pass: “YOUR_PASS”,
},
});
let info = await transporter.sendMail({
from: ‘”James Swanson” <[email protected]>’,
to: “[email protected]”,
subject: “I love SMTP!”,
text: “Here’s a text version of the email.”,
html: “Here’s an <strong>HTML version</strong> of the email.”,
});
console.log(“Message sent: %s”, info.messageId);
console.log(“View email: %s”, nodemailer.getTestMessageUrl(info));l
}
main().catch(console.error);
Note that we’ve also added your email address in the “to” field. It is the test email address that you can use to see whether the emails reach the destination inbox successfully.
Step 4. Go back to the terminal and run your application:
node nodemailer-smtp.js
If everything went well, you get a notification similar to the following and see the email in your test email address’ inbox:
Message sent: <[email protected]>
View email: https://ethereal.email/message/Yn08JBjWtKQFtVIQYn0-fjRYXoiZYQBGAAAAA.ty0T2jOGponZjUaXLFoWU
Congrats! Now you can send emails using Node.js and SMTP.
How to send emails with transactional email API
There are lots of services you can use for transactional email API setup that we listed earlier. Today, we’ll show how to send emails using SendGrid API.
Step 1. Sign up for SendGrid and create a SendGrid API key in the Settings menu. Name the key and, under Permissions, select Full Access. Then, by clicking Create & View, you can copy your API key.
Step 2. Install the SendGrid client using npm and create a file named sendgrip.js:
npm install –save @sendgrid/mail
touch sendgrid.js
Step 3. Add the following to the file you’ve just created:
const sendgrid = require(‘@sendgrid/mail’);
const SENDGRID_API_KEY = “<SENDGRID_API_KEY>”
sendgrid.setApiKey(SENDGRID_API_KEY)
const msg = {
to: ‘[email protected]’,
from: ‘[email protected]’,
subject: ‘Sending with SendGrid Is Fun’,
text: ‘and easy to do anywhere, even with Node.js’,
html: ‘<strong>and easy to do anywhere, even with Node.js</strong>’,
}
sendgrid
.send(msg)
.then((resp) => {
console.log(‘Email sent\n’, resp)
})
.catch((error) => {
console.error(error)
})
Step 4. Create a sender identity to verify your email address with SendGrid. Then, insert that email address in the “from” field in the code in Step 3.
Also, paste the correct recipient’s email address in the “to” field.
Lastly, replace the SENDGRID_API_KEY variable with the SendGrid API key you generated in Step 1.
Step 5. Test whether your app works by running your application in the terminal:
node sendgrid.js
If the email was delivered successfully, you will see a sent email notification in the Activity section on SendGrid’s dashboard, which you can find on the sidebar. You will be able to see whether the email was opened, too.
And that’s it — you can now send emails with Node.js using a transactional email API service.
Sending HTML emails
Nodemailer supports sending HTML emails; in fact, it’s very easy to send HTML emails with this module.
To create an HTML email in Node.js, just add the HTML attribute to the message:
message = {
from: “[email protected]”,
to: “[email protected]“,
text: ‘Hey there, it’s our first message sent with Nodemailer 😉 ‘,
html: ‘<b>Hey there! </b><br> This is our first message sent with Nodemailer’
};
There you go — now you have an HTML ready to be sent. Again, you can use the sendMail() method to send this email.
Creating an HTML template
Nodemailer also allows creating email templates that you can use later for bulk HTML emails. Let’s consider an example of how to create a template to welcome new users.
We are using pug, the default option, to install the template engine via npm:
npm install email-templates pug
Next, we need to create 2 different files, one for the subject and one for the HTML body of the template. To do this, run the following:
subject.pug:
= `Hi ${firstName} ${lastName}, happy to see you at My App!`
html.pug:
h1 Hello #{firstName} #{lastName}
p.
Welcome to My App! Now your test emails will be safe. We just need to make sure your account is real.
Please, click the button below and start using your account.
a(href=’https://example.com/confirmation’) Confirm!
Then, add the files we have just created to your app directory:
app.js
emails
welcome (the template name)
html.pug
subject.pug
text.pug
Be sure to add the text part of your message, otherwise, it will be created automatically.
And now, we can gather everything together to add transport. Note that we’re using the Mailtrap Email API to send our email based on an HTML template:
const Email = require(’email-templates’);
const email = new Email({
message: {
from: ‘[email protected]’
},
send: true,
transport: {
host: ‘smtp.mailtrap.io’,
port: 2525,
ssl: false,
tls: true,
auth: {
user: ‘1a2b3c4d5e6f7g’,
pass: ‘1a2b3c4d5e6f7g’
}
}
});
const people = [
{firstName: ‘Diana’, lastName: ‘One’},
{firstName: ‘Alex’, lastName: ‘Another’}
];
people.forEach((person) => {
.send({
template: ‘welcome’,
message: {
to: ‘[email protected]’
},
locals: person
})
.then(console.log)
.catch(console.error);
}).
As a result, you will get a preview of your message in the browser.
And that’s it, you have an email template you can edit to create complex dynamic emails using CSS, tables, media and other additions.
Sending emails with attachments
Nodemailer also enables sending emails with Node.js that contain attachments. In fact, you can send any kind of attachment with your emails, but make sure that the email service you use accepts them.
So to add an attachment to your email, start with specifying email configurations:
const mailConfigurations = {
from: [email protected]’,
to: [email protected]’,
subject: ‘Sending Email using Node.js’,
Text: ‘Attachments can also be sent using Nodemailer’,
Then, start adding the attachments:
attachments: [
Depending on their type, you’ll have to use different properties:
- a UTF-8 string as an attachment (besides a string, it can also be a buffer or a stream):
{
filename: ‘text.txt’,
content: ‘Hello, receiver!’
},
- a file that can be accessed from a path on your machine (it’s the best way to add large attachments):
{
path: ‘/home/mrtwinklesharma/Programming/document.docx’
},
{
filename: ‘license.txt’,
path: ‘https://raw.github.com/nodemailer/nodemailer/master/LICENSE‘
},
- a URL with a comment as an attachment:
{
url: ‘mylist.example.com’,
comment: ‘My new list!’
}
]
};
These are some of the most popular kinds of attachments you may need to use in your emails when you send them with Nodemailer and Node.js. You can add as many attachments as you need, but note that you have to separate each attachment with a comma.
To Sum Up
And there you have it — now you know how to send emails using Node.js with an SMTP server and a transactional email API service. Sending emails through Node.js is simple and versatile. Because there are several options you can choose from, it won’t be a challenge to find the one that meets your needs best.
Author: Sofiia Kasianenko. Software Engineer and Mailtrap Contributor. Sofiia is interested in information technologies across all industries, from marketing to healthcare. Besides writing, she is passionate about creativity, professional growth, fitness, and well-being.
We will be helpful for you to support us on Twitter (if it’s still alive), LinkedIn, Facebook, and ProductHunt!
About our company
At Flatlogic we develop React, Angular and Vue templates and give our most professional clients a special tool to generate CRUD applications by themself and save over 250 hours of the development lifecycle. During the last 7 years, we have successfully completed more than 40 big projects for startups and large enterprises. As a team, we always have a deep desire to help our clients.
We are listed among the Top 20 Web Development companies from Lithuania. Fill out the form on the right corner of the main page and feel free to ask! If you are Professional Developer, check out other articles:
- Builder.ai Collapse: Startup Red Flags and How to Avoid Them
- Introducing Flatlogic Community Template: Free, Core Features, Simplified License
- Top 5+ Benefits of Using an AI Software Engineer for Startups
- Native OpenAI Integration to Flatlogic AI Engineer
- Lovable vs. Bolt vs. Replit: Which AI app coding tool is best? [ 2025]
Comments