Being a developer requires you to have a number of skills and extensive knowledge of what you work with. This includes knowing how to code in multiple programming languages, and more specifically, how to write clean code in JavaScript as it is one of the most widely-used coding languages today.
Unfortunately, not every developer has the necessary skills and experience to consistently write clean code in JavaScript. Without further ado, here are the best tips to follow to write clean functions in JavaScript.
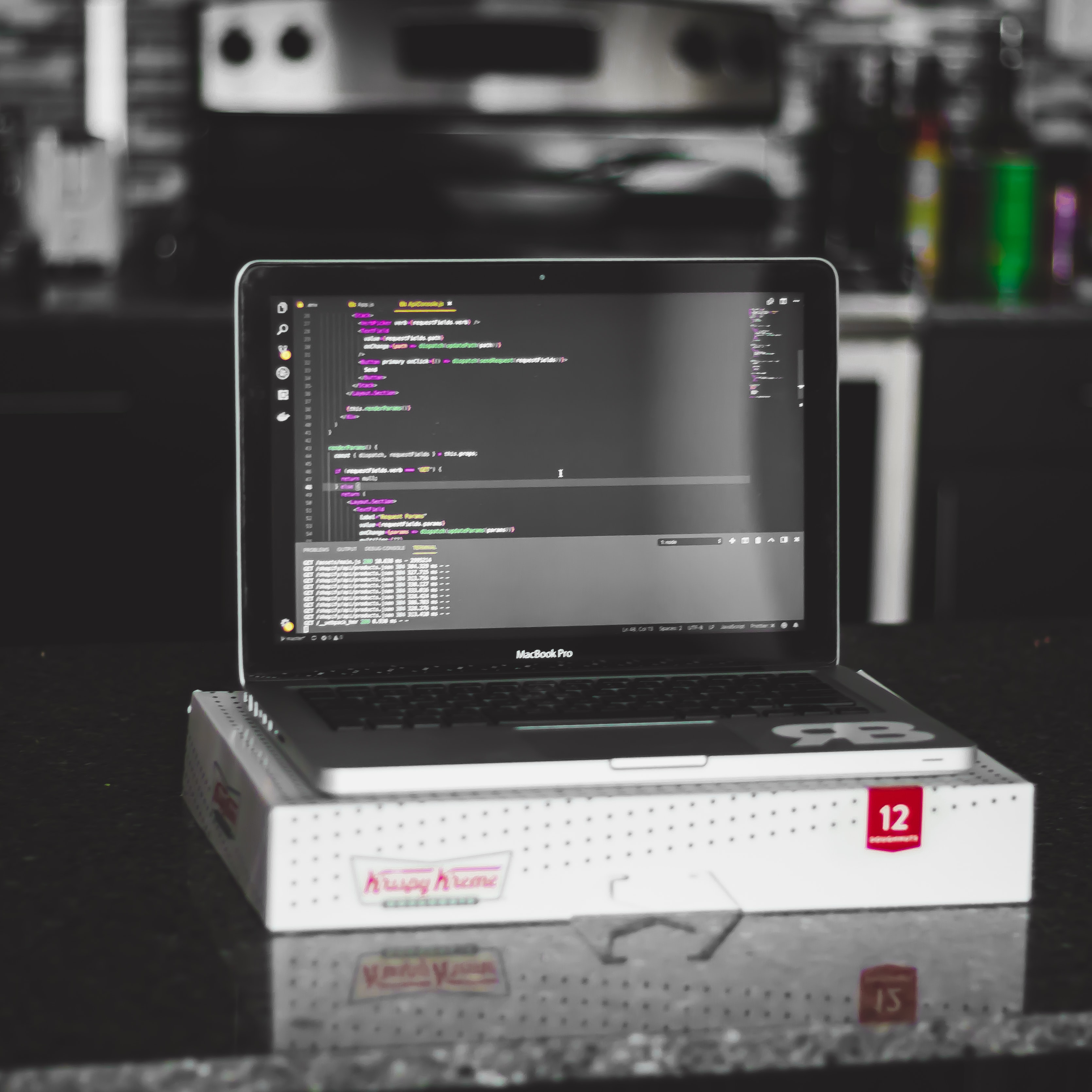
In Flatlogic we create web & mobile application templates built with React, Vue, Angular and React Native to help you develop web & mobile apps faster. Go and check out yourself!
See our themes!
#1 Know When to Take Your Mind Off Coding
Most of the tips in this article are technical in nature, but there are some tips that can help you change your mindset when coding which will improve your overall performance as a programmer. You need to know when to take your mind off coding – listen to podcasts for software developers, read interesting textbooks or industry journals, and so on. You can take such breaks while coding to reset your mind and then come back to your functions refreshed.
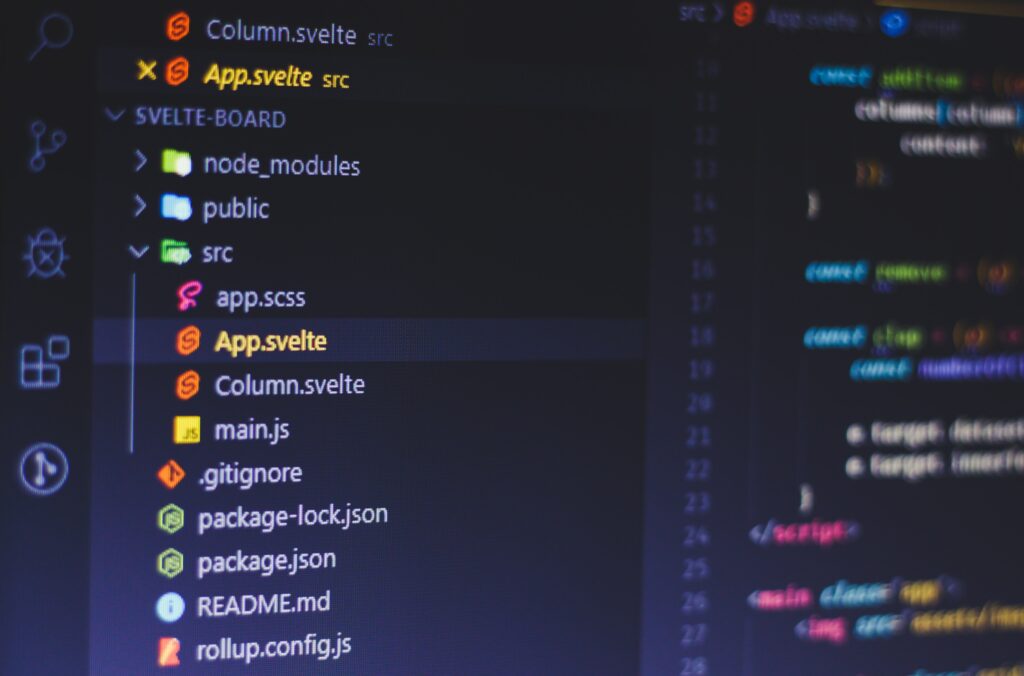
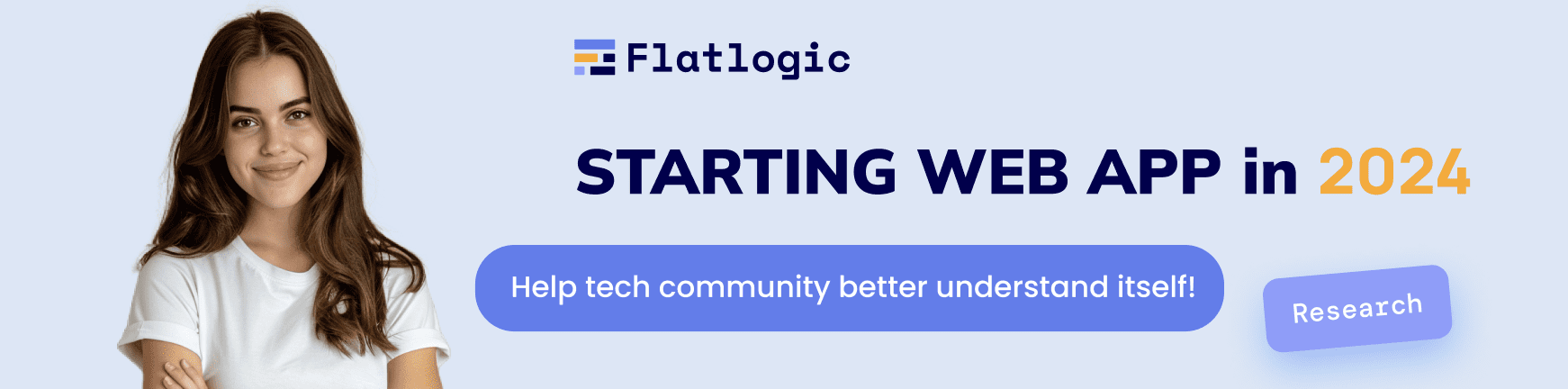
#2 Focus on Describing When Writing Code
The best way to write variables that you will instantly identify when you come back to coding after a break is the descriptive ones. Shorter names can look simpler and take up less space visually, but descriptive variable names will be clearer to you. This way, you won’t end up making mistakes or spending time trying to figure out which variable stands for what just because you took a break and forgot where you left off.
#3 Use Tools and Services to Help You
Some of the most efficient coders are the ones who use different tools and services to help them. This can be said for other professionals – for instance, content creators hire experienced writers from the writing services reviews site Rated by Students to help them write content. You can get similar assistance by using different software development tools and services that could help you in the coding process.\
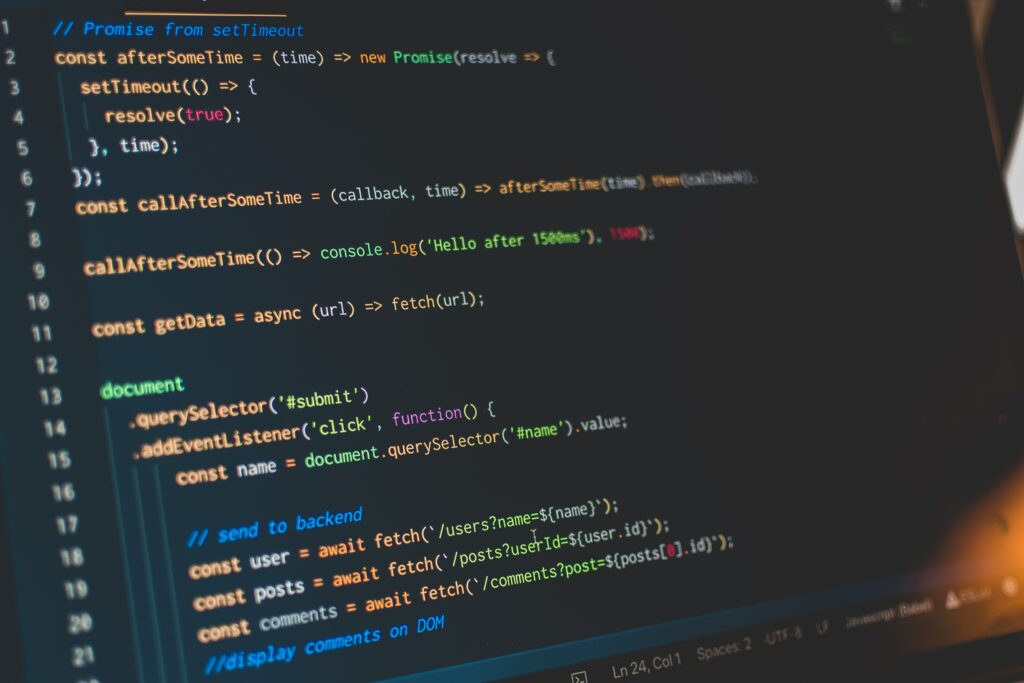
#4 Utilize Shorthand Wherever You Can
While making variable names longer by making them descriptive is essential, it doesn’t mean you can use shorter names for other things. In fact, it’s best to utilize shorthand wherever you can as it will save you both time and space. Here’s an example of how a function changes when you use shorthand:
// Original
if (x !== “” && x !== null && x !== undefined) { ... }
// Shorthand
if ( !!x ) { ... }
#5 Outsource to Maintain Productivity
As mentioned earlier, using tools and services is a good way to become more efficient at what you do. But in addition to that, you can also actively outsource some of your tasks while you focus on coding. You can hire an expert writer from the custom writing reviews site Top Writing Reviews to write reports or documents for you and find a virtual assistant who can schedule your meetings, send out emails, and so on. This way, you will be able to maintain productivity while coding because you won’t have to spend any energy on other tasks.
#6 Catch Up on the Latest Trends
The best writers at the most successful writing services are the ones who keep up with the trends. And the best programmers are the ones who keep up with JavaScript trends and other news in the industry. You don’t necessarily need to jump onto these trends, but you still need to know what they are at any given time and how you could use them in your own work if you decide to utilize them.
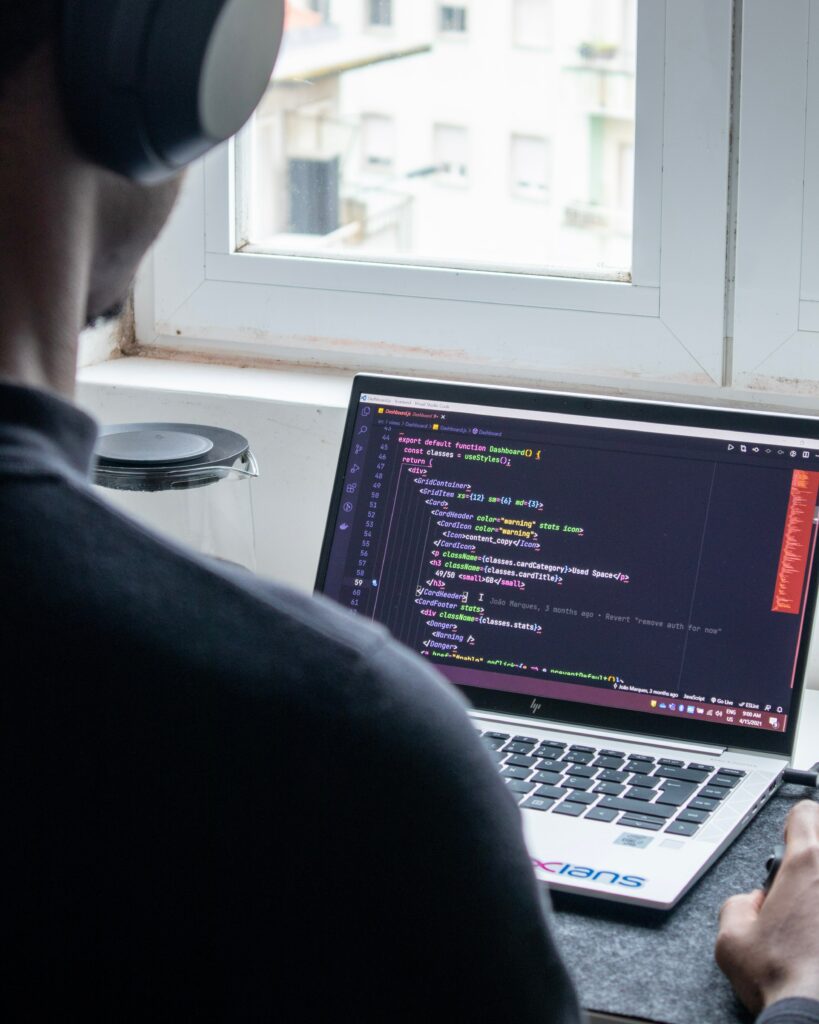
#7 Follow Common Best Practices
Instead of trying to invent something new, you can simply check the most common best practices used by JavaScript coders today and improve your skills as you get more experience. Here are the practices and principles you should start following:
#1 Object Destructuring
Object destructuring is used to take fields from an object and assign them to variables. This way, you will need fewer code lines to extract object properties. Moreover, your code will become much cleaner and easier to understand. You can use destructuring for cases with multiple object properties, the same property multiple times, and a property deep in an object.
#2 Arrow Functions
Utilizing arrow functions is perfect for writing cleaner code as this means that other elements like curly braces parenthesis become optional. You will be able to resolve the issue of accessing “this” property inside callbacks and write concise functions at the same time. Here’s an example of a regular function and an arrow function:
// Original
function myOrder(order){
console.log(
Customer need ${order}
);
}
// Arrow
const myOrder = order => console.log(`Customer need ${order}`);
#3 Multiple Parameters
Every time you are declaring a function, you need to use multiple input parameters instead of using single object inputs. By doing so, it will be easier to understand the minimum quantity of parameters that have to be passed by checking the method signature. This practice will also result in better application performance! That being said, make sure to switch to object parameters if the quantity of input parameters increases.
#4 Spread Extension Operator
The spread extension operator is a feature in ES6 that can be used to expand literals (e.g. arrays) into individual elements in the form of a single line of code. You can use this operator to put an array or object into a new array or object. You can also use it to combine multiple parameters in the array.
#5 Template Literals
Another tip is to use template literals (literals delimited with backticks) for string concatenations. With these, you can create multiline strings as well as perform string interpolation. An example of this principle in practice is defining a placeholder in a string in order to get rid of unnecessary concatenations:
// Original
var name = 'Peter';
var message = 'Hi '+ name + ',';
// New
var name = 'Peter';
var message = `Hi ${name},`;
#6 Avoid Callbacks
This practice is something you probably know about if you are up to date with the trends. Using callbacks used to be a popular technique for expressing and handling asynchronous functions. Now that ES6 and ES7 introduced solutions (Promises or Async/Await) for working with asynchronous functions, you no longer need to rely on callbacks and can make your code cleaner and simpler.
#7 Early Exit
When writing any kind of function, aim to exit it early. If there are any options for you to do this, you will notice that exiting functions early will make them more concise, saving you both time and space. Try to use fewer indentations, avoid unnecessary pieces of code, etc.
#8 For-Of Loops
There are different kinds of loops that you can use, including for-of, for-I, forEach, and for-in. However, unlike the other loops, for-of loops have some clear advantages that you can benefit from. These loops have fewer characters, are much easier to read, and even have the ability to continue, return, or break from the loop. This is what using for-of loops looks like in practice:
// Original
for (let i = 0; i < cars.length; i++) {
const car = cars[I];
}
// New
var name = 'Peter';
var message = `Hi ${name},`;
#9 String Interpolation
When writing code, aim to use string interpolation over concatenation. Doing so will make your code more readable to interpolate. Here is an example of how you can do this:
#10 Avoid Double Negatives
In some cases, double negatives can be very subtle, so you need to be particularly careful. Avoid them at all costs because they can easily lead to bugs along with making your code too convoluted.
#11 Maximum Two Arguments
Every time you work with arguments, you will need to order them in a logical way. Unfortunately, this is difficult when there are three or more arguments. This is why you need to try to avoid declaring functions that have more than two arguments.
#12 Use Try-Catch with Await
Rather than using a tree of .then() calls, try using async await. It will make your code much more readable and clean. However, keep in mind that the awaited values could throw rejections that you will then have to catch.
#13 Avoid “Magic” Numbers
Last but not least, avoid using “magic” numbers. Strings and numbers that have meanings that aren’t immediately obvious have to be declared as separate descriptive variables. Here is an example:
// Original
total += cost * 0.0375;
// New
const serviceFee = 0.0375;
total += cost * serviceFee
Final Thoughts
At the end of the day, the quality of your JavaScript functions depends entirely on you. If you make the effort to write clean code and use the tips above, you will definitely improve your skills and become a better specialist at your job.
Author: Nancy Howard
About blog
At Flatlogic we develop React, Angular and Vue templates and give our most professional clients a special tool to generate CRUD applications by themself and save over 250 hours of the development lifecycle. During the last 7 years, we have successfully completed more than 40 big projects for startups and large enterprises. As a team, we always have a deep desire to help our clients.
We will be helpful for you to support us on Twitter (if it’s still alive), LinkedIn, Facebook, and ProductHunt!
We are listed among the Top 20 Web Development companies from Lithuania. Fill out the form on the right corner of the main page and feel free to ask! If you are Professional Developer, check out other articles:
Comments